- Published on
Using Paramiko module in Python to execute Remote Bash Commands
- Authors
- Name
- Ruan Bekker
- @ruanbekker
Paramiko is a python implementation of the sshv2 protocol.
Paramiko to execute Remote Commands:
We will use paramiko module in python to execute a command on our remote server.
Client side will be referenced as (side-a) and Server side will be referenced as (side-b)
Getting the Dependencies:
Install Paramiko via pip on side-a:
$ pip install paramiko --user
Using Paramiko in our Code:
Our Python Code:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(hostname='192.168.10.10', username='ubuntu', key_filename='/home/ubuntu/.ssh/mykey.pem')
stdin, stdout, stderr = ssh.exec_command('lsb_release -a')
for line in stdout.read().splitlines():
print(line)
ssh.close()
Execute our Command Remotely:
Now we will attempt to establish the ssh connection from side-a, then run lsb_release -a
on our remote server, side-b:
$ python execute.py
Distributor ID: Ubuntu
Description: Ubuntu 16.04.4 LTS
Release: 16.04
Codename: xenial
Thank You
Thanks for reading, feel free to check out my website, and subscribe to my newsletter or follow me at @ruanbekker on Twitter.
- Linktree: https://go.ruan.dev/links
- Patreon: https://go.ruan.dev/patreon
Please feel free to show support by, sharing this post, making a donation, subscribing or reach out to me if you want me to demo and write up on any specific tech topic.
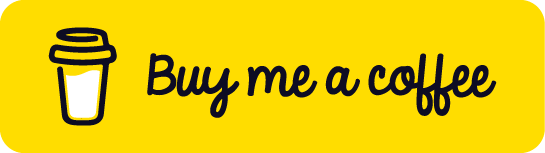