- Published on
Web Forms with Python Flask and the WTForms Module with Bootstrap
- Authors
- Name
- Ruan Bekker
- @ruanbekker
Quick demo with Web Forms using the WTForms module in Python Flask.
Requirements:
Install the required dependencies:
$ pip install flask wtforms
Application:
The Application code of the Web Forms Application. Note that we are also using validation, as we want the user to complete all the fields. I am also including a function that logs to the directory where the application is running, for previewing the data that was logged.
app.py
from random import randint
from time import strftime
from flask import Flask, render_template, flash, request
from wtforms import Form, TextField, TextAreaField, validators, StringField, SubmitField
DEBUG = True
app = Flask(__name__)
app.config.from_object(__name__)
app.config['SECRET_KEY'] = 'SjdnUends821Jsdlkvxh391ksdODnejdDw'
class ReusableForm(Form):
name = TextField('Name:', validators=[validators.required()])
surname = TextField('Surname:', validators=[validators.required()])
def get_time():
time = strftime("%Y-%m-%dT%H:%M")
return time
def write_to_disk(name, surname, email):
data = open('file.log', 'a')
timestamp = get_time()
data.write('DateStamp={}, Name={}, Surname={}, Email={} \n'.format(timestamp, name, surname, email))
data.close()
@app.route("/", methods=['GET', 'POST'])
def hello():
form = ReusableForm(request.form)
#print(form.errors)
if request.method == 'POST':
name=request.form['name']
surname=request.form['surname']
email=request.form['email']
password=request.form['password']
if form.validate():
write_to_disk(name, surname, email)
flash('Hello: {} {}'.format(name, surname))
else:
flash('Error: All Fields are Required')
return render_template('index.html', form=form)
if __name__ == "__main__":
app.run()
HTML Template:
templates/index.html
This will result in a basic web form like this:
Resources:
- https://getbootstrap.com/docs/4.1/components/alerts/
- https://pythonspot.com/en/download-flask-examples/
- https://flask-wtf.readthedocs.io/en/stable/quickstart.html
- https://pythonspot.com/flask-web-forms/
Thank You
Thanks for reading, feel free to check out my website, and subscribe to my newsletter or follow me at @ruanbekker on Twitter.
- Linktree: https://go.ruan.dev/links
- Patreon: https://go.ruan.dev/patreon
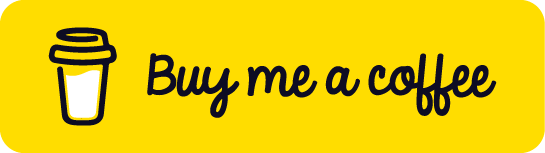