- Published on
Creating a UI in Python Flask and Bootstrap for our Serverless URL Shortener
- Authors
- Name
- Ruan Bekker
- @ruanbekker
From a previous post, we went through the setup of building a Serverless URL Shortener with API Gateway, Lambda, and DynamoDB on AWS. Today we will build a Web User Interface using Python Flask, Bootstrap and JavaScript that will communicate to our API to shorten URL's.
Note: Although using Python Flask is a Hosted option, you could also use this example to host it as a web page on Amazon S3, for the complete serverless route.
Dependencies:
We need Flask, Gunicorn (optional) and Requests:
$ pip install flask gunicorn requests
Application Code:
It's good practice to use a API Key for some level of security, but if not, you can just remove the headers section of x-api-key
.
The application relies on 3 environment variables: APP_TITLE
- which is the banner name (defaults to "My URL Shortener" if none is set), TINY_API_URL
- which is the URL to create the shortened url and X_API_KEY
which is the api key for your API.
The content of app.py
:
from flask import Flask, render_template, request, url_for
import os
import sys
import socket
import requests
import json
import logging
tiny_api_url = os.getenv('TINY_API_URL', None)
tiny_api_key = os.getenv('X_API_KEY', None)
app_title = os.getenv('APP_TITLE', 'My URL Shortener')
if tiny_api_url == None or tiny_api_key == None:
logging.error("Failed to load configuration")
sys.exit(4)
headers = {'Content-Type': 'application/json', 'X-Api-Key': tiny_api_key}
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html', app_title=app_title)
@app.route('/shortened', methods=['GET', 'POST'])
def search_request():
user_url = request.form["input"]
response = requests.post(
tiny_api_url,
headers=headers,
data=json.dumps({
"long_url": user_url
}
)
)
return render_template('results.html', app_title=app_title, res=response.content )
if __name__ == '__main__':
app.run(passthrough_errors=False)
JavaScript
We want to copy the value of the shortened url response to clipboard when clicking on a button. For that functionality, we need some javascript.
$ mkdir -p static/js
$ touch static/js/clipboard.js
the content for our javascript function - static/js/clipboard.js
:
function copyToClipboard() {
var copyText = document.getElementById("input");
copyText.select();
document.execCommand("Copy");
}
HTML
The content for templates/index.html
:
The content for templates/results.html
:
Run the Server
Before we run the server, we need to set the environment variables as mentioned earlier:
TINY_API_URL=https://tiny-api.mydomain.com/create
X_API_KEY=someRandomSecretKey09876543210
Run the Server:
$ gunicorn -w 2 -b 0.0.0.0:8080 --access-logfile=/dev/stdout --error-log=/dev/stderr app:app
After booting the server, access the server on http://localhost:8080/
and the response should look like:
Dockerizing this Application
The source code for this project is available on my github repository
Thank You
Thanks for reading, if you like my content, feel free to check out my website, and subscrube to my newsletter or follow me at @ruanbekker on Twitter.
- Linktree: https://go.ruan.dev/links
- Patreon: https://go.ruan.dev/patreon
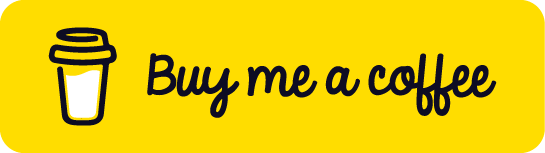