- Published on
MongoDB Examples with Golang
- Authors
- Name
- Ruan Bekker
- @ruanbekker
While looking into working with mongodb using golang, I found it quite frustrating getting it up and running and decided to make a quick post about it.
What are we doing?
Examples using the golang driver for mongodb to connect, read, update and delete documents from mongodb.
Environment:
Provision a mongodb server in docker:
$ docker network create container-net
$ docker run -itd --name mongodb --network container-net -p 27017:27017 ruanbekker/mongodb
Drop into a golang environment using docker:
$ docker run -it golang:alpine sh
Get the dependencies:
$ apk add --no-cache git
Change to your project path:
$ mkdir $GOPATH/src/myapp
$ cd $GOPATH/src/myapp
Download the golang mongodb driver:
$ go get go.mongodb.org/mongo-driver
Connecting to MongoDB in Golang
First example will be to connect to your mongodb instance:
package main
import (
"context"
"fmt"
"log"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/bson"
"go.mongodb.org/mongo-driver/mongo/options"
)
type Person struct {
Name string
Age int
City string
}
func main() {
clientOptions := options.Client().ApplyURI("mongodb://mongodb:27017")
client, err := mongo.Connect(context.TODO(), clientOptions)
if err != nil {
log.Fatal(err)
}
err = client.Ping(context.TODO(), nil)
if err != nil {
log.Fatal(err)
}
fmt.Println("Connected to MongoDB!")
}
Running our app:
$ go run main.go
Connected to MongoDB!
Writing to MongoDB with Golang
Let's insert a single document to MongoDB:
func main() {
..
collection := client.Database("mydb").Collection("persons")
ruan := Person{"Ruan", 34, "Cape Town"}
insertResult, err := collection.InsertOne(context.TODO(), ruan)
if err != nil {
log.Fatal(err)
}
fmt.Println("Inserted a Single Document: ", insertResult.InsertedID)
}
Running it will produce:
$ go run main.go
Connected to MongoDB!
Inserted a single document: ObjectID("5cb717dcf597b4411252341f")
Writing more than one document:
func main() {
..
collection := client.Database("mydb").Collection("persons")
ruan := Person{"Ruan", 34, "Cape Town"}
james := Person{"James", 32, "Nairobi"}
frankie := Person{"Frankie", 31, "Nairobi"}
trainers := []interface{}{james, frankie}
insertManyResult, err := collection.InsertMany(context.TODO(), trainers)
if err != nil {
log.Fatal(err)
}
fmt.Println("Inserted multiple documents: ", insertManyResult.InsertedIDs)
}
This will output in:
$ go run main.go
Inserted Multiple Documents: [ObjectID("5cb717dcf597b44112523420") ObjectID("5cb717dcf597b44112523421")]
Updating Documents in MongoDB using Golang
Updating Frankie's age:
func main() {
..
filter := bson.D{{"name", "Frankie"}}
update := bson.D{
{"$inc", bson.D{
{"age", 1},
}},
}
updateResult, err := collection.UpdateOne(context.TODO(), filter, update)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Matched %v documents and updated %v documents.\n", updateResult.MatchedCount, updateResult.ModifiedCount)
}
Running that will update Frankie's age:
$ go run main.go
Matched 1 documents and updated 1 documents.
Reading Data from MongoDB
Reading the data:
funct main() {
..
filter := bson.D{{"name", "Frankie"}}
var result Trainer
err = collection.FindOne(context.TODO(), filter).Decode(&result)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Found a single document: %+v\n", result)
findOptions := options.Find()
findOptions.SetLimit(2)
}
$ go run main.go
Found a single document: {Name:Frankie Age:32 City:Nairobi}
Finding multiple documents and returning the cursor
func main() {
..
var results []*Trainer
cur, err := collection.Find(context.TODO(), bson.D{{}}, findOptions)
if err != nil {
log.Fatal(err)
}
for cur.Next(context.TODO()) {
var elem Trainer
err := cur.Decode(&elem)
if err != nil {
log.Fatal(err)
}
results = append(results, &elem)
}
if err := cur.Err(); err != nil {
log.Fatal(err)
}
cur.Close(context.TODO())
fmt.Printf("Found multiple documents (array of pointers): %+v\n", results)
}
Running the example:
$ go run main.go
Found multiple documents (array of pointers): [0xc0001215c0 0xc0001215f0]
Deleting Data from MongoDB:
Deleting our data and closing the connection:
func main(){
..
deleteResult, err := collection.DeleteMany(context.TODO(), bson.D{{}})
if err != nil {
log.Fatal(err)
}
fmt.Printf("Deleted %v documents in the trainers collection\n", deleteResult.DeletedCount)
err = client.Disconnect(context.TODO())
if err != nil {
log.Fatal(err)
} else {
fmt.Println("Connection to MongoDB closed.")
}
}
Running the example:
$ go run main.go
Deleted 3 documents in the trainers collection
Connection to MongoDB closed.
The code for this example can be found at github.com/ruanbekker/code-examples/mongodb/golang/examples.go
Resources:
Thank You
Thanks for reading, if you like my content, feel free to check out my website, and subscribe to my newsletter or follow me at @ruanbekker on Twitter.
- Linktree: https://go.ruan.dev/links
- Patreon: https://go.ruan.dev/patreon
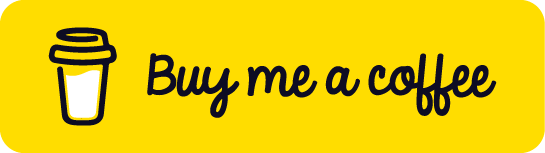