- Published on
How to Create a KinD Kubernetes Terraform Module
- Authors
- Name
- Ruan Bekker
- @ruanbekker
Deploying Kubernetes clusters locally is a great way to test and develop your applications without the need for cloud resources. In this blog post, we will walk you through creating a Terraform module to deploy a Kubernetes cluster using the Kind (Kubernetes in Docker) provider. This guide is perfect for developers and DevOps engineers looking to streamline their local Kubernetes setup process.
Prerequisites
Ensure you have the necessary tools and dependencies installed before getting started.
Required Tools:
- Docker
- Terraform
- Kubectl
Published Terraform Module
If you are interested in the code, the module has been published to my Github repository:
Directory Structure
The directory structure looks like the following:
├── main.tf
├── outputs.tf
├── providers.tf
└── variables.tf
Explanation of each file:
main.tf
: The main Terraform configuration fileoutputs.tf
: Definitions of output variablesproviders.tf
: The provider configurationvariables.tf
: Definitions of input variables
First we will show the main.tf
, and one important part is the kubeconfig_path
, which is where the provider will write the kube config yaml configuration to. We can set the KUBECONFIG
environment variable to this path afterwards to access our cluster.
resource "kind_cluster" "this" {
name = var.cluster_name
node_image = "kindest/node:${var.cluster_version}"
kubeconfig_path = pathexpand(var.kubeconfig_file)
wait_for_ready = true
kind_config {
kind = "Cluster"
api_version = "kind.x-k8s.io/v1alpha4"
node {
role = "control-plane"
extra_port_mappings {
container_port = 80
host_port = var.host_port
}
}
node {
role = "worker"
}
}
}
Then our outputs.tf
:
output "endpoint" {
value = kind_cluster.this.endpoint
}
output "client_certificate" {
value = base64encode(kind_cluster.this.client_certificate)
}
output "client_key" {
value = base64encode(kind_cluster.this.client_key)
}
output "cluster_ca_certificate" {
value = base64encode(kind_cluster.this.cluster_ca_certificate)
}
output "kubeconfig" {
value = kind_cluster.this.kubeconfig
}
Then for our providers.tf
:
terraform {
required_providers {
kind = {
source = "tehcyx/kind"
version = "0.5.1"
}
}
}
And lastly our variables.tf
:
variable "cluster_name" {
type = string
default = "test"
description = "The kubernetes cluster name."
}
variable "cluster_version" {
type = string
default = "v1.27.1"
description = "The kubernetes version."
}
variable "host_port" {
type = number
default = 18080
description = "The host port to be bound to port 80."
}
variable "kubeconfig_file" {
type = string
default = "/tmp/kube.config"
description = "The file location for kubeconfig content."
}
Deploying the Kubernetes Cluster
Steps to initialize and apply the Terraform configuration to deploy the Kind cluster.
First we need to create our directory where we want to source our module from:
mkdir example
Then we create our example/main.tf
with the following:
module "kubernetes" {
source = "../"
# source = "git::https://github.com/ruanbekker/terraform-kubernetes-kind-module.git?ref=main"
cluster_name = "test-cluster"
cluster_version = "v1.27.1"
kubeconfig_file = "/tmp/kube.config"
}
Now that we have pointed our source to our terraform module, we can initialize terraform, which will download the module and its deoendencies:
cd example
terraform init
Now we can deploy our infrastructure, by running:
terraform apply -auto-approve
The module have written the kubeconfig to the value of our kubeconfig_file
variable that was configured, now we can tell our kubectl client where to access the configuration:
export KUBECONFIG=/tmp/kube.config
Now we can use the kubectl client and test access to the cluster:
kubectl get nodes
Conclusion
Summarize the steps taken and discuss the benefits of using Terraform and Kind for local Kubernetes clusters.
Key Takeaways
- Simplified local Kubernetes deployments
- Repeatable and version-controlled infrastructure
- Flexibility for development and testing
Resources
The following resources can be used for reference:
- https://github.com/ruanbekker/terraform-kubernetes-kind-module
- https://ruan.dev/blog/2022/09/20/kind-for-local-kubernetes-clusters
Thank You
Thanks for reading, if you like my content, feel free to check out my website, and subscribe to my newsletter or follow me at @ruanbekker on Twitter.
- Linktree: https://go.ruan.dev/links
- Patreon: https://go.ruan.dev/patreon
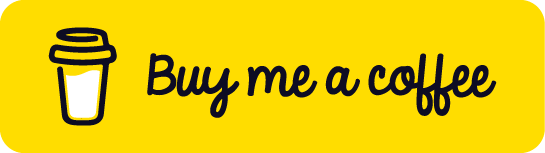